Retirement Calculator Python Program
Retirement Calculator. Ages are in years. Def retirement_calculator(current_age, yearly_contribution=0, current_savings=0, retirement_age=65, avg_annual_return=1.07).
How can I find an age in python from today's date and a persons birthdate? The birthdate is a from a DateField in a Django model.
16 Answers
That can be done much simpler considering that int(True) is 1 and int(False) is 0:
In Python 3, you could perform division on datetime.timedelta
:
As suggested by @[Tomasz Zielinski] and @Williams python-dateutil can do it just 5 lines.
Unfortunately, you cannot just use timedelata as the largest unit it uses is day and leap years will render you calculations invalid. Therefore, let's find number of years then adjust by one if the last year isn't full:
Upd:
This solution really causes an exception when Feb, 29 comes into play. Here's correct check:
Upd2:
Calling multiple calls to now()
a performance hit is ridiculous, it does not matter in all but extremely special cases. The real reason to use a variable is the risk of data incosistency.
The classic gotcha in this scenario is what to do with people born on the 29th day of February. Example: you need to be aged 18 to vote, drive a car, buy alcohol, etc ... if you are born on 2004-02-29, what is the first day that you are permitted to do such things: 2022-02-28, or 2022-03-01? AFAICT, mostly the first, but a few killjoys might say the latter.
Here's code that caters for the 0.068% (approx) of the population born on that day:
Here's the output:
Here is a solution to find age of a person as either years or months or days.
Lets say a person's date of birth is 2012-01-17T00:00:00Therefore, his age on 2013-01-16T00:00:00 will be 11 months
or if he is born on 2012-12-17T00:00:00, his age on 2013-01-12T00:00:00 will be 26 days
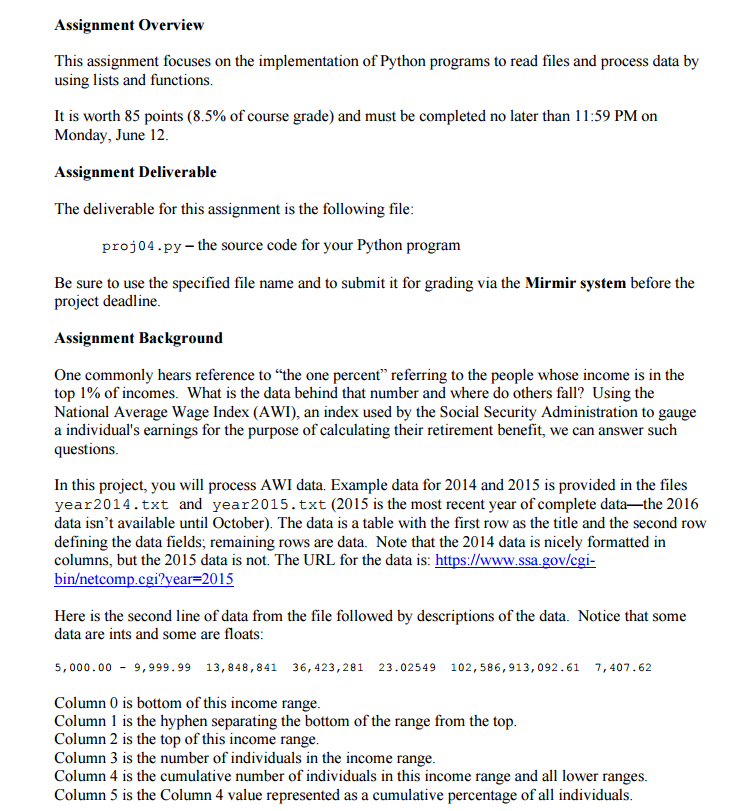
or if he is born on 2000-02-29T00:00:00,his age on 2012-02-29T00:00:00 will be 12 years
You will need to import datetime.
Here is the code:
Some extra functions used in the above codes are:
And
Now, we have to feed get_date_format() with the strings like 2000-02-29T00:00:00
It will convert it into the date type object which is to be fed to get_person_age(date_birth, date_today).
The function get_person_age(date_birth, date_today) will return age in string format.
If you're looking to print this in a page using django templates, then the following might be enough:
Expanding on Danny's Solution, but with all sorts of ways to report ages for younger folk (note, today is datetime.date(2015,7,17)
):
Sample code:
As I did not see the correct implementation, I recoded mine this way...
Assumption of being '18' on the 28th of Feb when born on the 29th is just wrong.Swapping the bounds can be left out ... it is just a personal convenience for my code :)
Extend to Danny W. Adair Answer, to get month also
Slightly modified Danny's solution for easier reading and understanding
protected by Community♦Feb 10 '14 at 12:53
Thank you for your interest in this question. Because it has attracted low-quality or spam answers that had to be removed, posting an answer now requires 10 reputation on this site (the association bonus does not count).
Would you like to answer one of these unanswered questions instead?
Not the answer you're looking for? Browse other questions tagged pythondatetimedate or ask your own question.
The code below, albeit somewhat messy, asks the user to input their: years until retirement, interest rate, initial amount, and amount added each year. That part works fine. What I am trying to do now is calculate the value every five years based on the user's input. Basically I want it to look something like this:
The code I have now does mostly that except I think I either have a math error or a coding error (or both) that I can't seem to figure out. Any help would be greatly appreciated. My code is below:
My current program outputs something like this:
1 Answer
change this to :
this:
In your case you taken range(5,year_left+5), it will generarate a lit of number from 5 to year_left+5, so it will be like [5,6,7,8,9.....year_left+5]
, it not going to increment by 5.
more pythonic will be:
it will go by 5 increment